Leveraging the vSphere API for Automated VM Configuration Management
As VMware administrators, we often face the challenge of efficiently managing and monitoring our virtual infrastructure. While the vSphere Client provides a user-friendly interface for manual operations, there are compelling reasons to embrace the vSphere API for automation.
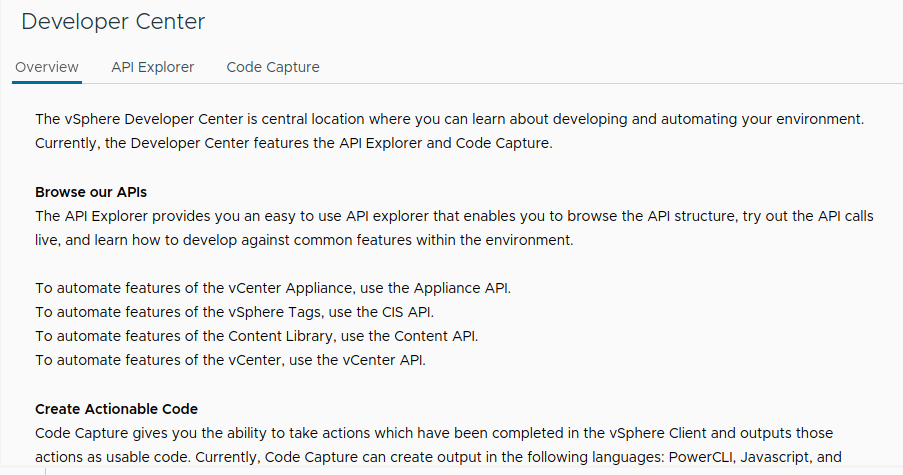
In this post, we'll explore why you should consider using the vSphere API and break down a practical script that helps you collect VM configuration data automatically.
What does the script in this blog post do? It will connect to the vSphere REST API and then collect the virtual machines and their configuration settings. Eventually the script exports the data to a csv file.
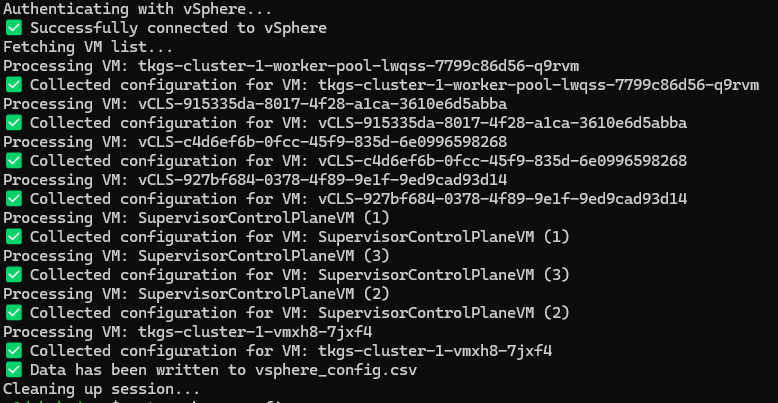
The Bash script
The Script
First, let's create our environment variables.
export VCENTER_SERVER='your-vcenter-server'
export VCENTER_USER='your-username'
export VCENTER_PASSWORD='your-password'
Now, here's our main script. Create a file called vsphere-monitor.sh
:
#!/bin/bash
# Exit on error
set -e
# Check if required environment variables are set
if [ -z "$VCENTER_SERVER" ] || [ -z "$VCENTER_USER" ] || [ -z "$VCENTER_PASSWORD" ]; then
echo "❌ Please set all required environment variables:"
echo " VCENTER_SERVER, VCENTER_USER, VCENTER_PASSWORD"
exit 1
fi
# Output file
OUTPUT_FILE="vsphere_config.csv"
# Function to handle errors
handle_error() {
echo "❌ Error: $1"
exit 1
}
# Function to cleanup session
cleanup() {
if [ ! -z "$SESSION_ID" ]; then
echo "Cleaning up session..."
curl -k -s -X DELETE \
-H "vmware-api-session-id: $SESSION_ID" \
"https://$VCENTER_SERVER/api/session" || true
fi
}
# Set trap for cleanup
trap cleanup EXIT
# Get session ID
echo "Authenticating with vSphere..."
SESSION_ID=$(curl -k -s -X POST \
-u "${VCENTER_USER}:${VCENTER_PASSWORD}" \
"https://$VCENTER_SERVER/api/session" \
-H 'Accept: application/json' \
| tr -d '"') || handle_error "Failed to authenticate"
if [ -z "$SESSION_ID" ]; then
handle_error "Failed to get session ID"
fi
echo "✅ Successfully connected to vSphere"
# Initialize CSV file
echo "timestamp,vm_name,power_state,cpu_count,memory_size_mb,disk_capacity_gb" > "$OUTPUT_FILE"
# Get list of VMs
echo "Fetching VM list..."
VM_LIST=$(curl -k -s \
-H "vmware-api-session-id: $SESSION_ID" \
"https://$VCENTER_SERVER/api/vcenter/vm" \
-H 'Accept: application/json') || handle_error "Failed to get VM list"
# Process each VM
echo "$VM_LIST" | jq -r '.[] | @base64' | while read -r vm_info; do
# Decode VM info
VM_ID=$(echo "$vm_info" | base64 -d | jq -r '.vm')
VM_NAME=$(echo "$vm_info" | base64 -d | jq -r '.name')
POWER_STATE=$(echo "$vm_info" | base64 -d | jq -r '.power_state')
echo "Processing VM: $VM_NAME"
# Get VM details
VM_DETAILS=$(curl -k -s \
-H "vmware-api-session-id: $SESSION_ID" \
"https://$VCENTER_SERVER/api/vcenter/vm/$VM_ID" \
-H 'Accept: application/json') || continue
# Extract configuration details
CPU_COUNT=$(echo "$VM_DETAILS" | jq -r '.cpu.count')
MEMORY_MB=$(echo "$VM_DETAILS" | jq -r '.memory.size_MiB')
# Calculate total disk capacity
DISK_GB=$(echo "$VM_DETAILS" | jq -r '
[.disks | to_entries[] | .value.capacity] |
add / (1024 * 1024 * 1024) |
round |
tostring
')
# Get current timestamp
TIMESTAMP=$(date '+%Y-%m-%d %H:%M:%S')
# Write to CSV
echo "$TIMESTAMP,$VM_NAME,$POWER_STATE,$CPU_COUNT,$MEMORY_MB,$DISK_GB" >> "$OUTPUT_FILE"
echo "✅ Collected configuration for VM: $VM_NAME"
done
echo "✅ Data has been written to $OUTPUT_FILE"
Why Use the vSphere API?
- Automation at Scale: Manual configuration and monitoring become impractical as your infrastructure grows. The vSphere API allows you to manage hundreds or thousands of VMs programmatically.
- Consistency and Reliability: Human error is eliminated when operations are automated through API calls. Your scripts will perform the same way every time they run.
- Integration Capabilities: The API enables seamless integration with other tools and systems in your infrastructure, making it possible to build comprehensive automation workflows.
- Time Efficiency: Tasks that would take hours to complete manually can be executed in minutes through automated API calls.
- Audit and Compliance: API-based solutions make it easier to track changes, generate reports, and maintain compliance records.
Understanding the VM Configuration Collection Script
Let's break down a practical example: a Bash script that uses the vSphere API to collect configuration details from all VMs and export them to a CSV file.
Key Components
1. Environmental Security
if [ -z "$VCENTER_SERVER" ] || [ -z "$VCENTER_USER" ] || [ -z "$VCENTER_PASSWORD" ]; then
echo "❌ Please set all required environment variables:"
echo " VCENTER_SERVER, VCENTER_USER, VCENTER_PASSWORD"
exit 1
fi
The script uses environment variables for sensitive credentials, following security best practices by keeping credentials out of the code.
2. Session Management
SESSION_ID=$(curl -k -s -X POST \
-u "${VCENTER_USER}:${VCENTER_PASSWORD}" \
"https://$VCENTER_SERVER/api/session" \
-H 'Accept: application/json' \
| tr -d '"')
The script establishes a session with the vSphere API, storing the session ID for subsequent requests. It includes proper cleanup through a trap mechanism to ensure sessions are terminated properly.
3. Data Collection
The script collects essential VM information including:
- VM name
- Power state
- CPU count
- Memory size
- Total disk capacity
4. Error Handling
handle_error() {
echo "❌ Error: $1"
exit 1
}
Robust error handling ensures the script fails gracefully when encountering issues, making it suitable for production environments.
5. Output Format
The script generates a CSV file with timestamps, making it perfect for:
- Historical tracking
- Capacity planning
- Configuration management
- Compliance reporting
Using the Script
- Set your environment variables:
export VCENTER_SERVER="vcenter.yourdomain.com"
export VCENTER_USER="administrator@vsphere.local"
export VCENTER_PASSWORD="your-password"
- Run the script:
chmod +x vm_config_collector.sh
The script will create a CSV file containing configuration details for all VMs in your environment.
Best Practices
When working with the vSphere API:
- Always Clean Up Sessions: The script demonstrates proper session cleanup using trap commands.
- Handle Errors Gracefully: Implement comprehensive error handling for production use.
- Secure Credentials: Use environment variables or secure vaults rather than hardcoding credentials.
- Rate Limiting: Consider implementing rate limiting for large environments to avoid overwhelming the vCenter Server.
Conclusion
This script provides a quick and efficient way to inventory your vSphere environment using just bash and common Unix tools. It's particularly useful for:
- Regular configuration auditing
- Capacity planning
- Documentation requirements
- Integration with other bash-based tools
You can extend this script to collect additional metrics or modify the output format to suit your needs.